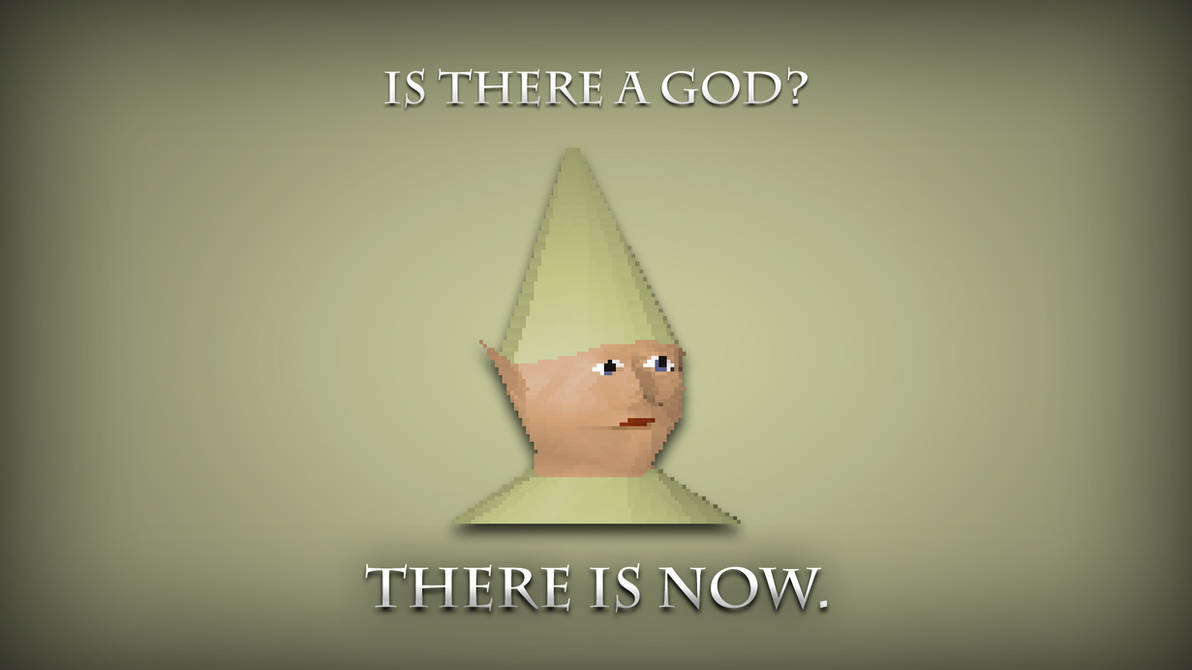
🍄
Ibán site
This Blog/Site Is Provided 'As Is' Without Warranty of Any Kind.
About me:
- Iván Molina Rebolledo
- /dev/méxico
- In the bottom of the sea.
- Github: https://github.com/ivanmoreau
- Email: [email protected]
- BlueSky Social - Bsky: https://bsky.app/profile/ivanmoreau.bsky.social
- UNAM (National Autonomous University of Mexico) dropout
- I like functional programming
Ex Senior Software Engineer at https://github.com/IvanAtDealEngine, and during my leisure time, I pursue my passion for magic (doing software things, music, literature or things like that I enjoy).